How to build a typewriter with JavaScript
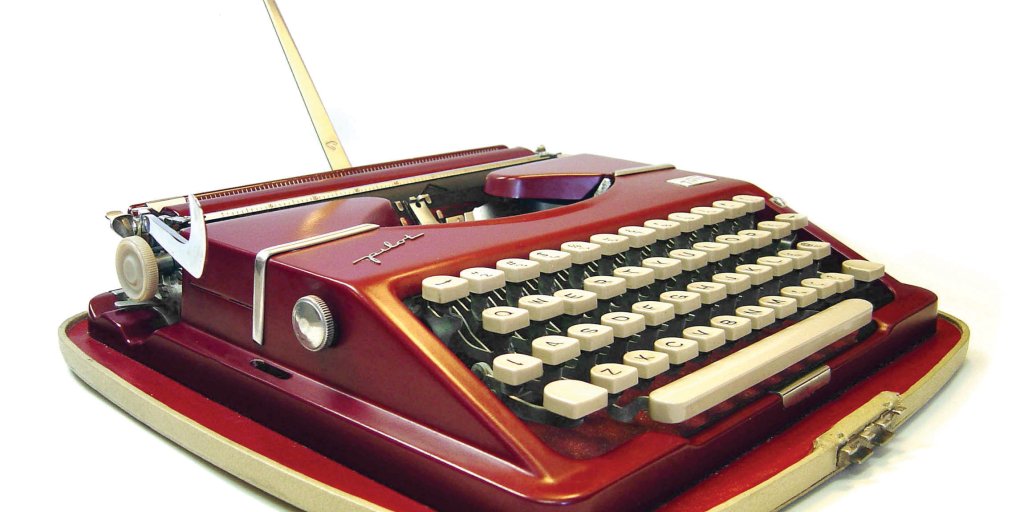
Img source: time.com
This article is also available on hashnode
If you have wandered around the web, you may have came across some very simple nice animated type-writers on some websites. Some are very complex. In this tutorial, we are going to build a very simple typewriter with vanilla JavaScript.
Create 3 files. if you are using Mac or Linux run
mkdir typewriter
and
cd typewriter.
However if you
are not on Mac/Linux you can manually create this files.
To know that you are currently in the typewriter directory, type
pwd
in the terminal and
it will output home/user/typewriter$
. Now type this in
your terminal touch index.html style.css script.js
this
will create 3 files specified in the command. To actually see your
files type ls
in the terminal.
This is all we need for the setup. The entire code for the tutorial is
available in the pen below. Copy and paste it in appropriate files
you've created.
See the Pen typewriter by RK (@KanhaFly) on CodePen.
What did we do here?
Here I will mostly just focus on explaining the code in the
script.js
file. The entire code in the HTML and CSS3 files
is simple but if you don't understand please consider learning more
about HTML and CSS. We created a string containing the
text to be written called textToWrite
and grabbed onto
our paragraph tag with an id of text
in our html
file and asigned it to a variable called textElem
.
To actually start
writting our text to the paragraph in our HTML file, we created a function
writer
. We then write text to the paragraph element using
the innerHTML
method and the slice
method to
extract characters from our string variable. Remember we're keeping Track
of the current character with a dummy global variable currentChar
which we declared outside our function and assigned it a value of 0
and incremented it inside our function(currentChar += 1
).
How the slice
String method works.
The JavaScript string slice() method is used to fetch the part of the string and returns the new string. It is required to specify the index number as the start and end parameters to fetch the part of the string. The index starts from 0.
This method allows us to pass a negative number as an index. In such case, the method starts fetching from the end of the string. It doesn't make any change in the original string.
Syntax
string.slice(start, end)
start
- It represents the position of the string from where the fetching starts.
end
- It is optional. It represents the position up to which the string fetches. In other words, the end parameter is not included.
It returns part of a string.
The simpleconditional
statement in there just checks if the currentChar is greater than the length of a string and assign it a value of 0.
To actually start our writer function, we have to invoke it. We invoke it inside a
setInterval
method.
How the setInterval
method works.
The setInterval() method calls a function or evaluates an expression at specified intervals (in milliseconds).
Note
: 1000 ms = 1 second.
Syntax
setInterval(function, milliseconds, param1, param2, ...)
function
Required. The function that will be executed.
milliseconds
Required. The intervals (in milliseconds) on how often to execute the code. If the value is less than 10, the value 10 is used
param1, param2, ...
Optional. Additional parameters to pass to the function.
As soon as we call the setInterval method, the writer function will start displaying text sequentially in the document.
Conclusion
We've just build a simple typewriter with JavaScript. We have learned how to use different methods such as innerHTML
, slice
, and how the setInterval
function works. You can play around with the code to build more fun things.